binary tree python library
This becomes tree with only a root node. Even though Pythons standard library doesnt contain a premade solution weve seen here that to create a binary tree with Python one needs little more than a single custom class with a few helpers methods.
Python Binary Tree Implementation Python Guides
Let us suppose we have a binary tree and we need to check if the tree is balanced or not.
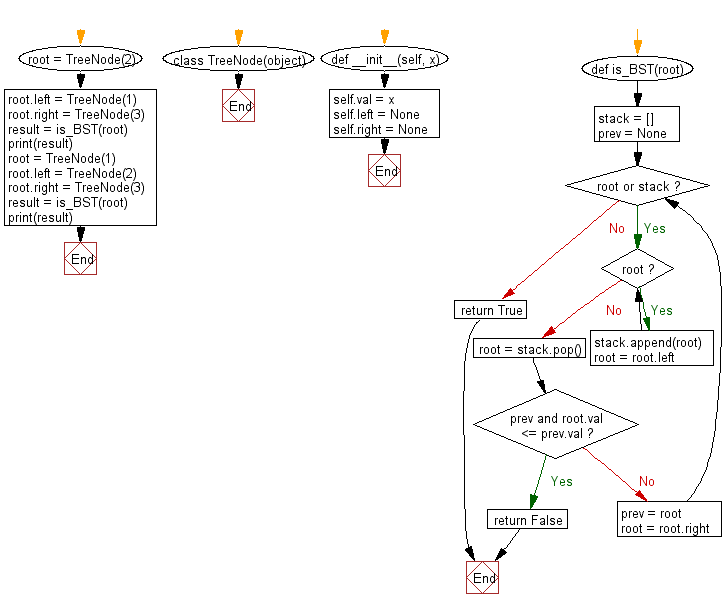
. Binarytree is Python library which lets you generate visualize inspect and manipulate binary trees. In this post well look at how to use Pythons dstructure module to create a binary tree and. The dstructure package is a Python library for data structures and algorithms.
Binarytree is a Python library which provides a simple API to generate visualize inspect and manipulate binary trees. Trees can be uses as drop in replacement for dicts in most cases. Skip the tedious work of setting up test data and dive straight into practising algorithms.
IntroductionA Python implementation of binary tree. Each node being a data component one a left child and the other the right child. Firstly we need to import from binarytree import Node and the node class represents the structure of a particular node in the binary tree.
In Python a binary tree can be represented in different ways with different data structuresdictionary list and class representations for a node. The attribute of this class are values left right. Def __init__ self data.
Library to draw binary trees in Python and Ruby. Basically its extended version of linked list. It also supports heap and binary search treeBST.
It allows you to skip the tedious work of setting up test data and dive straight into practising your algorithms. There are various concepts related to binary trees like different ways to traverse a binary tree the left and right view etc. A binary tree is comprised of nodes.
Binary tree is special type of heirarichal data structures defined using nodes. Heaps and BSTs binary search trees are also supported. The root node is the parent component on each subtree.
A Binary Tree is a non-linear data structure that is used for searching and data organization. A node is made up of three entities. Binarytree is a Python library which provides a simple API to generate visualize inspect and manipulate binary trees.
Python - Binary Tree Create Root. Pip install dstructure Binary Tree. It allows you to skip the tedious work of setting up test data and dive straight into practising your algorithms.
Creation of Binary Tree built using List. Its a tree data structure where each node is allowed to have maximum two children node generally referred as Left Child and Right Child. This module does not come pre-installed with Pythons standard utility module.
Python 27 or 34 Installation. Binary Search Trees are particularly useful for sorting and searchingstrongly hinted by the name. Binary tree is a widely-asked topic in coding contests and interviews.
Im trying to draw some binary trees in Python and Ruby and I was wondering if I can do it in Python with Tkinter or SymPy. While actual tress has its root at the bottom and all branches and leaves are on the top a binary tree has a root that sits on the top and all other branches and leafs are on the. The first node of the tree is left empty for better mathematical calculations.
In python a Binary tree can be implemented with the use of the list data structure as well. Creation of Node Constructor. Selfdata data selfleftChild None selfrightChild None def insertroot newValue.
In a binary tree each node contains two children ie left child and right child. Inserting into a Tree. To get the output I have used print Binary tree root.
This Classes are much slower than the built-in dict class but all iteratorsgenerators yielding data in sorted key order. A Binary tree is said to be balanced if the difference of height of left subtree and right subtree is less. Some basic knowledge of Python.
What Im trying to do is to show it in a window or save it to a file any of those would work. Heaps and binary search trees are also supported. In this article we will discuss how we can implement a binary tree and perform various operations using the dstructure library in Python.
Heaps and BSTs binary search trees are also supported. If binary search tree is empty create a new node and declare it as root if root is None. Let us dive into the concepts related to trees and implement them into the Python programming language.
Here we can see python binary tree implementation. To install dstructure open the terminal and write the below command. Since each element in a binary.
We can insert data. If the node does not have any value the user can set a value and return it. In Ruby I have absolutely no idea on how to do it.
However from your comments it sounds like you may have some other options. A binary tree is a set of finite nodes that can be empty or may contain several elements. We just create a Node class and add assign a value to the node.
It can also be considered as the topmost node in a tree. Root BinaryTreeNodenewValue return root if newValue is less than value of data in root add it to left subtree and proceed recursively if. Balanced Binary Tree in Python.
Python Server Side Programming Programming. No there is not a balanced binary tree in the stdlib. A value with two pointers on the left and right.
Python 27 or 34 Installation. However binarytree library helps to directly implement a binary tree. To implement the above structure we can define a class and assign values to data and reference to left child and right child.
This package provides Binary- RedBlack- and AVL-Trees written in Python and CythonC. To initialize a binary tree using a list we first create an empty list to store the nodes of the tree. A tree whose elements have at most two children is called a binary tree.
Binary tree in Python Step 1. A binary tree is a data structure also known as ordered tree is a rooted tree with a hierarchical structure reassembling an actual tree. If searching is all you need and your data are already sorted the bisect module provides a binary search algorithm for lists.
We need to create a Node class for Binary tree declaration. You say that you want a BST instead of a list for O log n searches. To insert into a tree we use the same node class created above and add a insert class to it.
Making Data Trees In Python Learn About Trees And How To Implement By Keno Leon The Startup Medium
Python Binary Tree Implementation Python Guides
Dynamically Constructing A Binary Tree Using Node Structure In Python Stack Overflow
Binary Search Tree Implementation 101 Computing
Height Of A Binary Tree Python Code With Example Favtutor
Implement A Tree Data Structure In Python Delft Stack
Binary Tree Methods In Python Kevin Vecmanis
Python Library For Creating Tree Graphs Out Of Nested Python Objects Dicts Stack Overflow
Coding Short Inverting A Binary Tree In Python By Theodore Yoong Medium
Python Binary Search Tree Bst Check A Binary Tree Is Valid Or Not W3resource
Python Tree Learn Trees In Data Structure With Examples
Machine Learning Are There Any Libraries For Non Binary Decision Trees In Python Stack Overflow
Plot Tree Plotting In Python Stack Overflow
Binary Search Trees With Python Level Up Coding
Level Order Traversal Of Binary Tree Python Code Favtutor
Level Order Traversal Of Binary Tree Python Code Favtutor
Machine Learning Are There Any Libraries For Non Binary Decision Trees In Python Stack Overflow